Block specific exact content with Adblock Plus : Youtube, LinkedIn, … stupid suggested content
As you can know we (software developers) are facing serious information overdose everyday, especially on social networks and video services such as Youtube.
On Youtube, like on Twitter, etc…. , you can both found shitposts and useful informations. This is a serious problem because we can easily waste time by filtering ourself everyday theses informations which take energy and time. This is a real problem.
Youtube : the grid of stupid videos
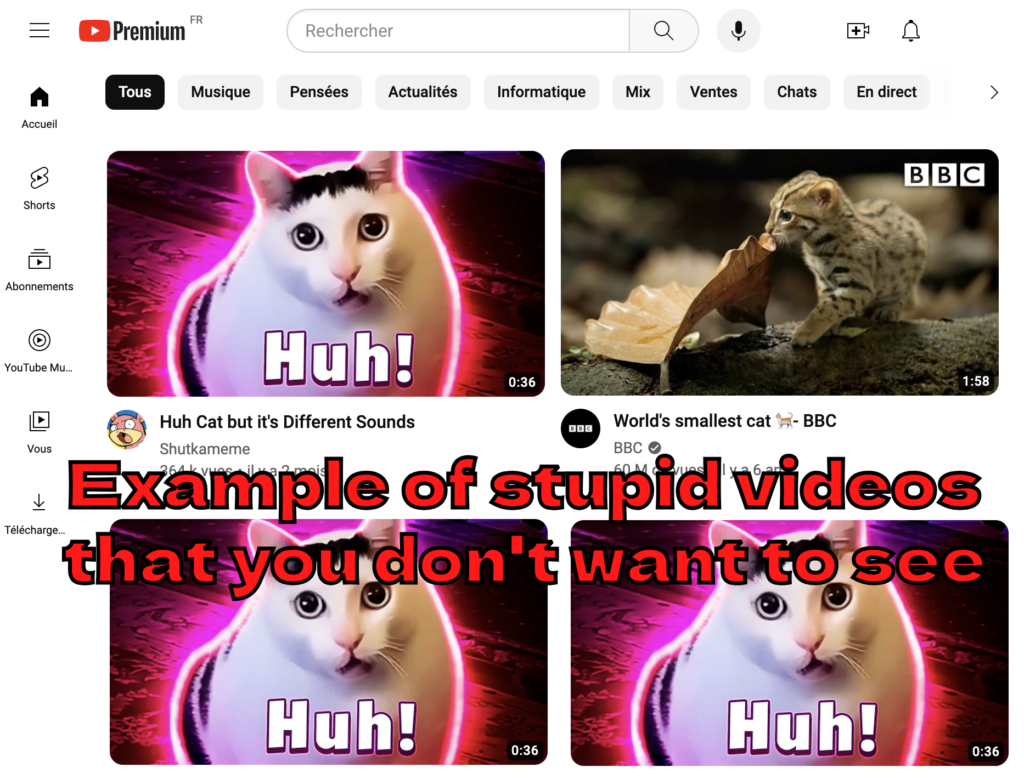
We can block the main Youtube page with add-ons like Adblock Plus (using others are possible but I will use this one in this tutorial).
As you can know, the social networks have psychologists and they are working hard with complex algorithms to waste your time and so you can be more on their platform and generate more money mainly with advertisement.
You can still see it if it is not a problem for you. As for me, the content is not relevant at all because watching cat videos will not help me about business, software development, earning money, and so on.
The solution
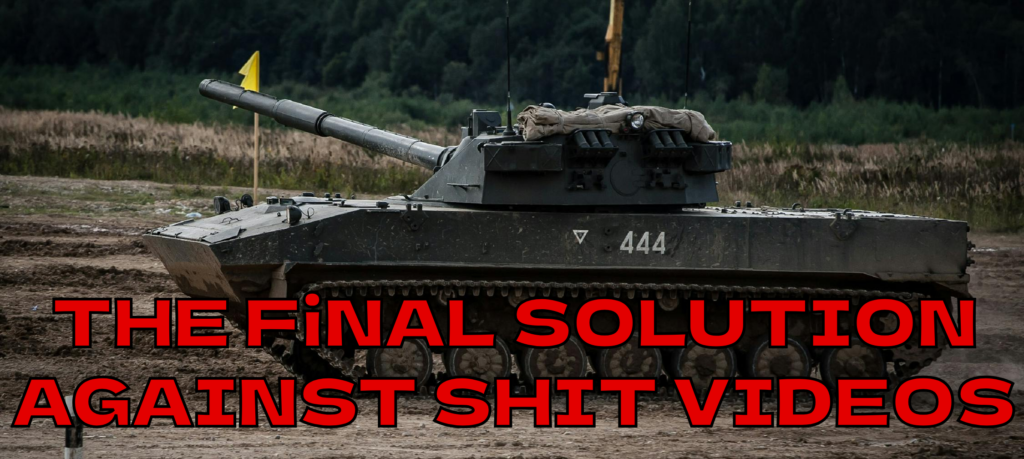
On Adblock Plus we can go to the Advanced settings :
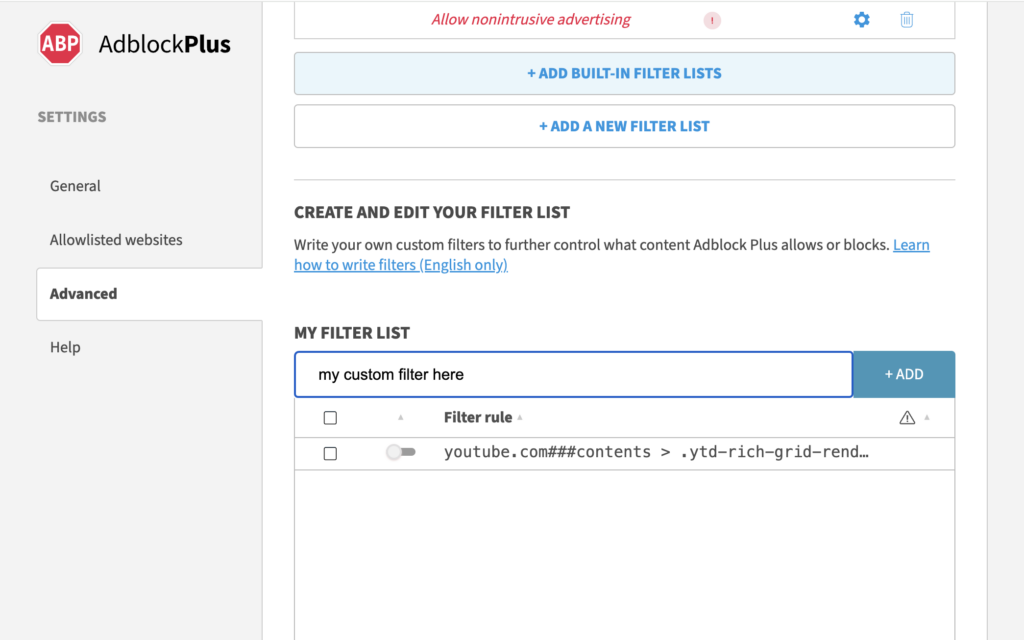
In “My Filter List” you can add filters that can block only the part of the website that contains useless informations.
Here is the Adblock Plus Cheatlist : https://adblockplus.org/filter-cheatsheet
We can now add the following rules to block the contents DIV
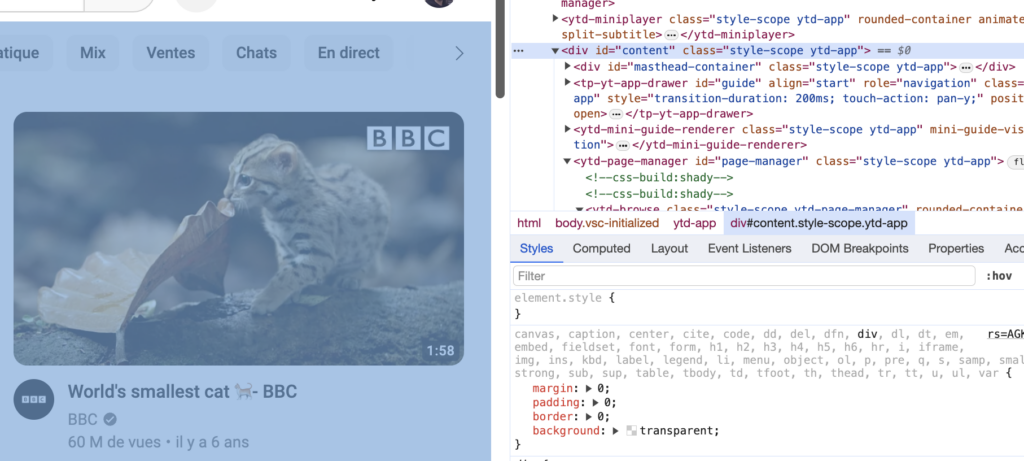
So, we can try to add the following filter:
youtube.com###contents
The problem if you use the rule is that you will not be able to a Youtube search:
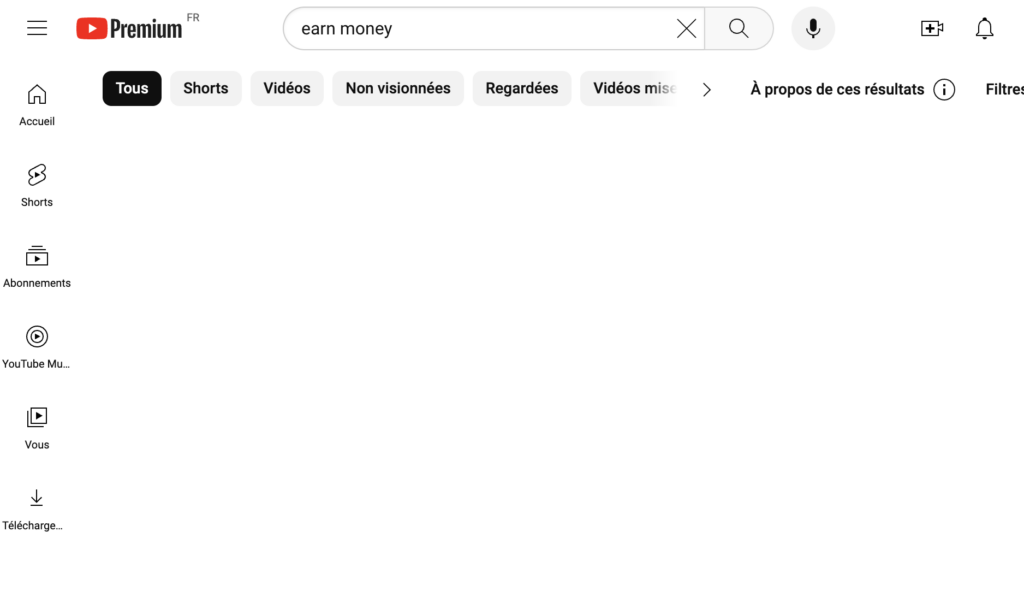
Now, if we go deeper in inspecting the DOM, we can see that:
- On the main page the DIV contents contains the class ytd-rich-grid-renderer if it is the main page
- On the search page the DIV contents contains the class ytd-section-list-renderer (and others)
If you look on the documentation of Adblock Plus, on the cheatlist, you can see that you can also block the ID and the CLASS, which will give the following rule:
youtube.com###contents > .ytd-rich-grid-renderer
The result:
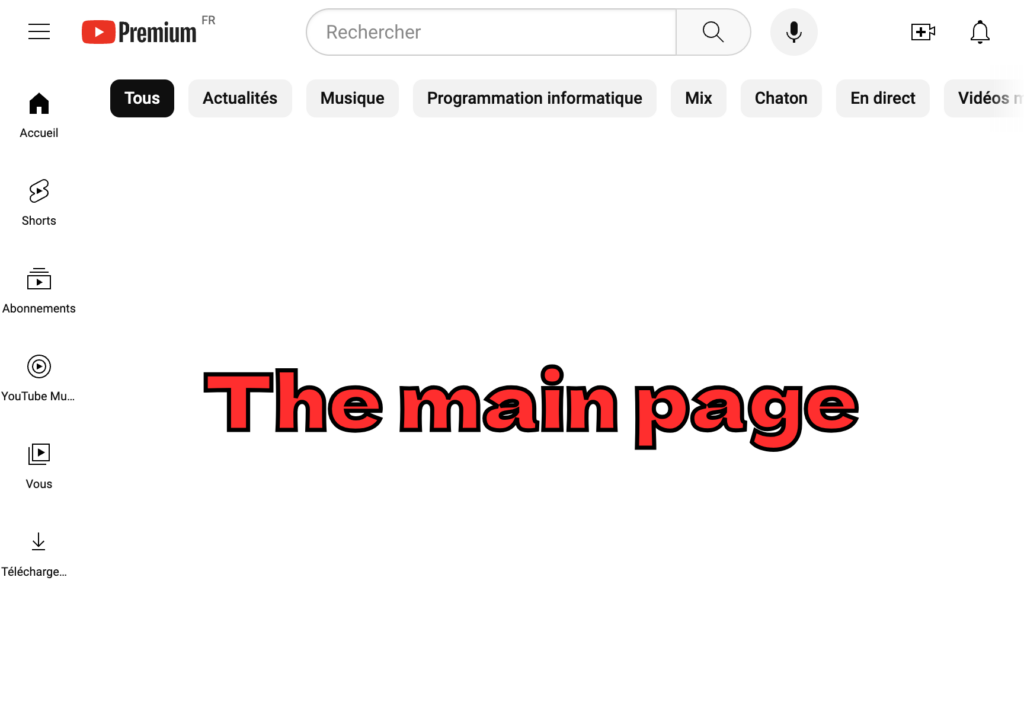
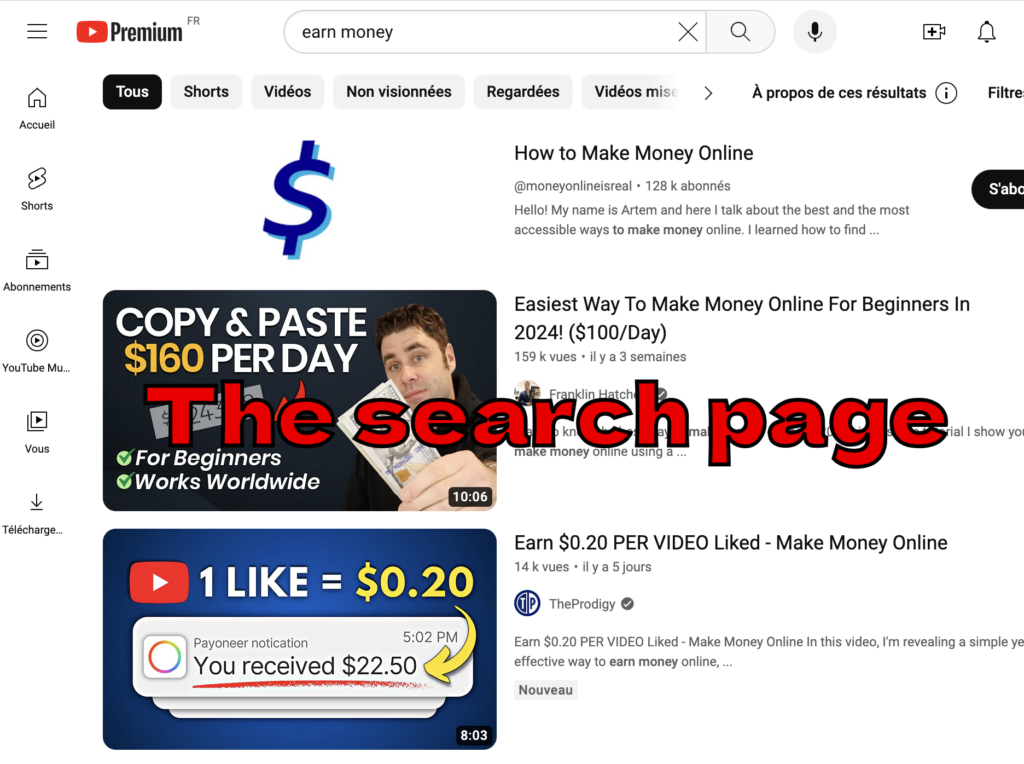
LinkedIn : the infinite scrolling
linkedin.com##.scaffold-finite-scroll
If you have others you can tell me ! 😃
Thank you for reading my article, do not hesitate to support me by contacting me or making a donation.