React.JS • Full list of all icons
Examples of listing the icons in React.JS / React Native
Listing all the icons
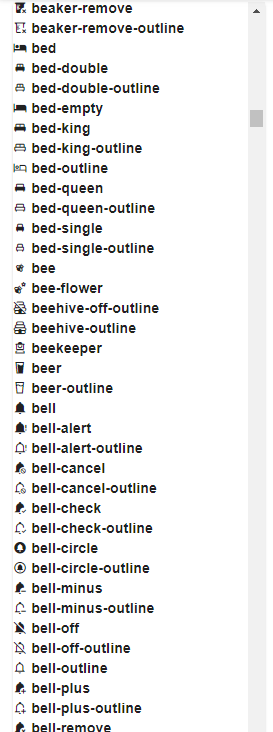
Searching icons name
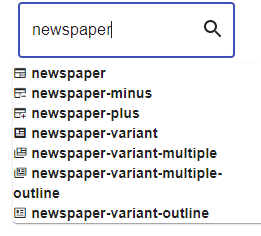
How to do it ?
Importing all the icons
Import the CSS your icons in /public/index.html
<link rel="stylesheet" href="http://cdn.materialdesignicons.com/5.4.55/css/materialdesignicons.min.css" />
Use it in your source code
Use your icons in the code source in /src/App.js like that:
<span>
<i class={`mdi mdi-${icon?icon:''}`} aria-hidden="true"></i>
</span>
Loop on all icons
If you want a list of icons then you can loop on the list of icons, let’s say you have put all your icons in a file called iconlist.js
export const icons = [
"ab-testing",
"abjad-arabic",
"abjad-hebrew",
"abugida-devanagari",
"abugida-thai",
"access-point",
"access-point-check",
"access-point-minus",
"access-point-network",
"access-point-network-off",
"access-point-off",
"access-point-plus",
"access-point-remove",
"account",
"account-alert",
"account-alert-outline",
"account-arrow-left",
"account-arrow-left-outline",
"account-arrow-right",
"account-arrow-right-outline",
"account-box",
"account-box-multiple",
"account-box-multiple-outline",
"account-box-outline",
"account-cancel",
"account-cancel-outline",
"account-cash",
"account-cash-outline",
"account-check",
...
];
Then you can import the icons in you App.js and use it:
import { icons } from './icons';
...
{ icons.map((icon, index) => (
<div style={{fontWeight: 'bold'}}> <i class={`mdi mdi-${icon}`} aria-hidden="true"></i> {icon} </div>
))}
You can then add a filter if you want to check that the icons contain part of the string name :
const [searchIcon, setSearchIcon] = useState(null);
...
{ icons.filter(icon => icon.includes(searchIcon) ).map((icon, index) => (
<div style={{fontWeight: 'bold'}}> <i class={`mdi mdi-${icon}`} aria-hidden="true"></i> {icon} </div>
)
)}