ReactJS • Responsive UI interface
You are making a React application and you want to have a responsive user interface that works both on laptop and mobile phone ?
A solution can be to use Material UI with several layout components such :
- Container
- Grid
- Hidden
The grid looks like this:
<Grid container spacing={3}>
<Grid item xs={12}>
<Paper className={classes.paper}>xs=12</Paper>
</Grid>
<Grid item xs={6}>
<Paper className={classes.paper}>xs=6</Paper>
</Grid>
<Grid item xs={6}>
<Paper className={classes.paper}>xs=6</Paper>
</Grid>
<Grid item xs={3}>
<Paper className={classes.paper}>xs=3</Paper>
</Grid>
<Grid item xs={3}>
<Paper className={classes.paper}>xs=3</Paper>
</Grid>
<Grid item xs={3}>
<Paper className={classes.paper}>xs=3</Paper>
</Grid>
<Grid item xs={3}>
<Paper className={classes.paper}>xs=3</Paper>
</Grid>
</Grid>
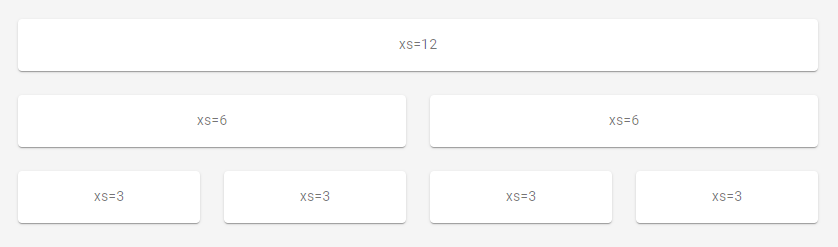
As you can see, the full width of the page is xs=12. It means the full width size is equal to 12.
You can see so that with 2 grid items of xs=6, it will be two components.
And so 4 will be 3 components on the line and 3 will be 4 components on the line.
You can use this grid items when you want to display block of contents on the page, if you know Bootstrap, it’s the same.
You can put any content inside your <Grid>, it can be a <div> for example.
Using XS, SM, MD, LG and XL
Grid Size | Device | Web browser width |
---|---|---|
XS | Mobile phone screens | 0px ► 600x |
SM | Mobile phone and tablets | 600px ► 960px |
MD | Laptop | 960px ► 1280px |
LG | Laptop | 1280px ► 1920px |
XL | Desktop | 1920px ► … |
Using breakpoints
Using breakpoints allow you to have different grid size for different devices, which allows you to have a grid adapted for mobile phone for exemple:
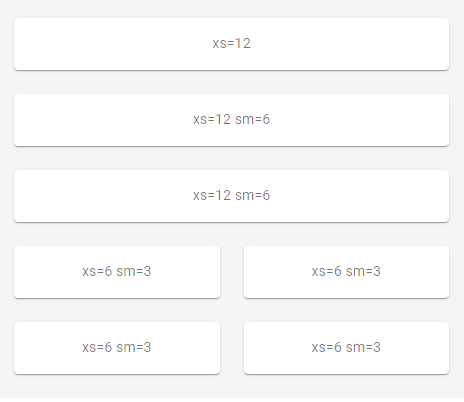
Using Hidden
You can use the <Hidden> element from Material UI to hide specific items for XS/SM/MD/LG/XL items.
<Hidden only="lg">
<Paper className={classes.paper}>Hidden on lg</Paper>
</Hidden>