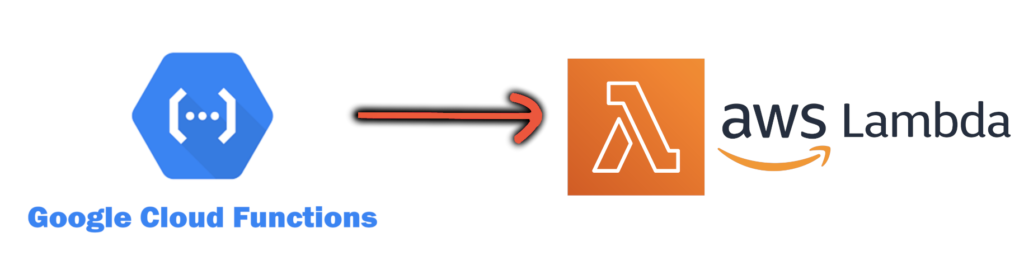
There are some differences between Google Cloud and AWS when you want to deploy your function for your API.
My point of view is that Google Cloud function may be a bit more simple to deploy but AWS is in a way the most reliable and complete cloud environnement nowadays.
On both cloud environnement you can upload the code with a ZIP, you can so use the same way to adjust your code for AWS:
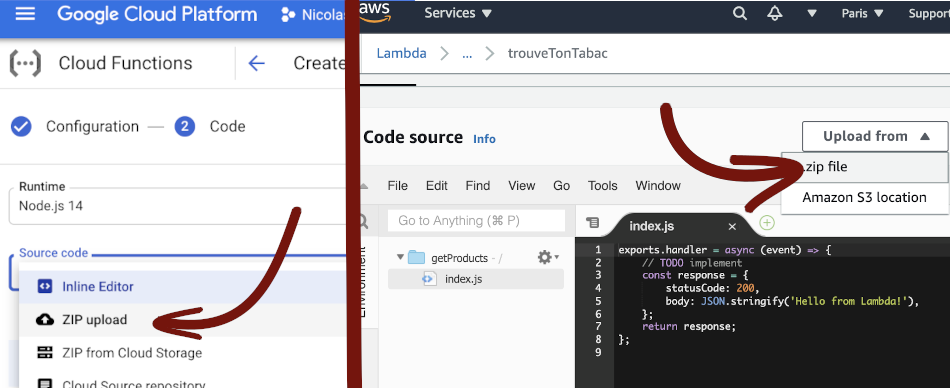
Differences between Google Cloud functions and AWS Lambda (Node.JS)
THE DEPENDENCIES
– In Google Cloud, the dependencies are automatically installed from the package.json file
– In AWS, you need to install the dependencies with npm or yarn, that will build the node_modules folder
If you don’t have the node_modules folder, your Lamdba function will not work with the following error:
{
"errorType": "Runtime.ImportModuleError",
"errorMessage": "Error: Cannot find module 'MODULE'\nRequire stack:\n- /var/task/index.js\n- /var/runtime/UserFunction.js\n- /var/runtime/index.js",
"trace": [
"Runtime.ImportModuleError: Error: Cannot find module 'MODULE'",
...
THE MAIN FUNCTION

With Google Cloud, the example function looks like this:
exports.helloWorld = (req, res) => {
res.status(200).send(message);
};
- You use the res object with the send() method to send the response

With AWS, the example function looks like this:
exports.handler = async (event) => {
const response = {
statusCode: 200,
body: JSON.stringify(jsonObj),
};
return response;
};
- You return a JSON object response that should contains the body and the statusCode
So the same code will looks like this:
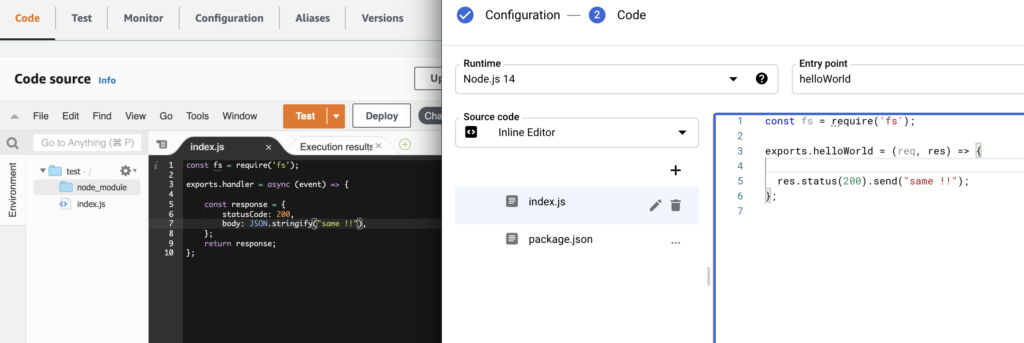
Using parameters
Let’s say now we want to use a parameter for our route, that will be number and the value will be 10, so we will call the route helloWorld?number=10

On GOOGLE CLOUD, to get the “number” parameter, we will use the req object :
exports.helloWorld = (req, res) => {
let number = req.query.number;

On AWS, to get the “number” parameter, we will use the event object :
exports.handler = async (event) => {
let number = event["params"]["number"];