Alerting user with a small messaga bubble – Snackbar – React.JS
In your web app, you will have to notice the user when he is doing some actions in your user interface. We can do it with a small message bubble called Snackbar, here is what it looks like:
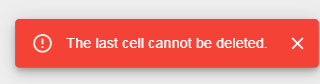
How we will do it
setSnackSeverity("error");
setSnackMessage("The last cell cannot be deleted.");
setOpenError(true);
In your code, we will use these 3 lines to:
– Set the severity “error”, there are 4 types of severity
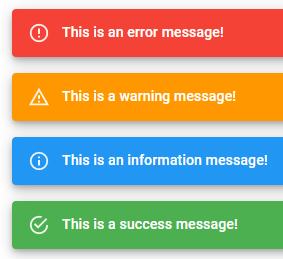
– Set the error message that will be displayed “The last cell cannot be deleted.”
– Show the error message (during few seconds)
Full example
import Snackbar from '@material-ui/core/Snackbar';
import MuiAlert, { AlertProps } from '@material-ui/lab/Alert';
function Alert(props) {
return <MuiAlert elevation={6} variant="filled" {...props} />;
}
...
const [snackSeverity, setSnackSeverity] = useState('error');
const [openError, setOpenError] = useState(false);
const [snackMessage,setSnackMessage] = useState('Error');
...
setSnackSeverity("error");
setSnackMessage("The last cell cannot be deleted.");
setOpenError(true);
...
<Snackbar
anchorOrigin={{ vertical: 'bottom', horizontal: 'right' }}
open={openError}
onClose={(e)=>setOpenError(false)}
autoHideDuration={3000}
>
<Alert onClose={(e)=>setOpenError(false)} severity={snackSeverity}>
{snackMessage}
</Alert>
</Snackbar>